Testing
Testing is a critical phase in the development process. This guide will help you ensure the reliability and functionality of your Tulu Switch integration by covering various testing methods and providing code examples.
Testing in a local environment
Learn how to set up and run your Tulu Switch integration in a local environment for effective testing before deploying to production.
Using Postman for API testing
Explore how to leverage Postman, a popular API testing tool, to validate your API requests and responses.
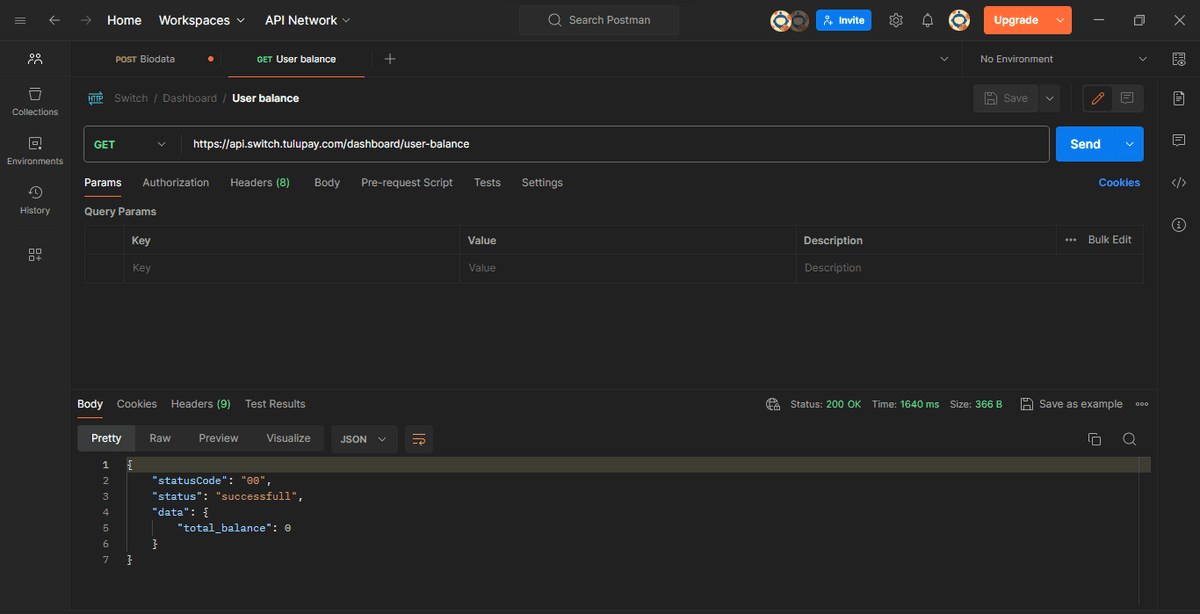
Unit testing (JavaScript, Python and PHP example):
Perform unit tests on your JavaScript, Python and PHP functions to verify their individual functionalities. Here's an example using Jest and React Testing Library:
// Sample React component
function MyComponent() {
// Component logic...
return <div>Hello, Tuluswitch!</div>;
}
// Jest unit test
test('renders component correctly', () => {
render(<MyComponent />);
expect(screen.getByText('Hello, Tuluswitch!')).toBeInTheDocument();
});
Integration testing (Generic HTTP example):
Conduct integration tests simulating HTTP requests to your Tulu Switch API. Here's a generic example using cURL:
// Sample integration test using Fetch API
test('integration test for HTTP GET request', async () => {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
expect(response.status).toBe(200);
expect(data).toHaveProperty('key');
});
End-to-end testing
E2E testing helps ensure that a system performs consistently and reliably under real-world conditions. It tests the system's ability to handle expected user loads and data volumes.
Load testing
Explore the various load testing tools and resources available to simulate real-world user traffic and optimize your system's performance. Remember, a little stress test now can save you a lot of heartache down the road.
Mocking Tulu Switch API responses
Discover the benefits of mocking, including faster development cycles, isolated testing of your code, and the ability to predict and manage API interactions effectively.
Contact Us for Developer Support
Contact our dedicated developer support team for assistance, guidance, or any questions you have regarding Tuluswitch API integration. We're here to ensure your development experience is smooth and successful.
Read Our Developer FAQ for Insights
Master the Tuluswitch API with our Developer Blog! Get expert insights, advanced tips, and industry trends beyond the documentation. Stay connected with the developer community to build exceptional payment platforms. Visit the blog now and unlock your full potential!